Mastering JavaScript: Extracting Specific Object Properties
Written on
Chapter 1: Understanding Object Property Subsets
In JavaScript, it’s often necessary to extract specific properties from an object. This article explores various methods to achieve that.
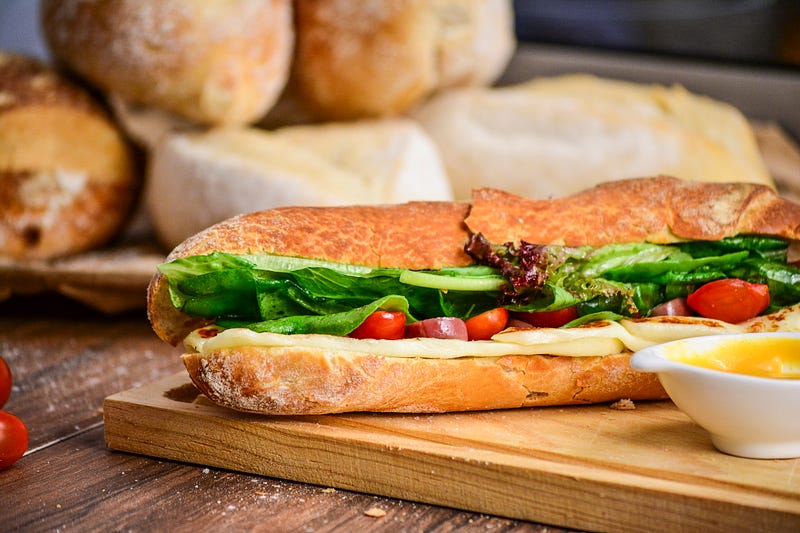
Section 1.1: Using Object Destructuring
One of the simplest and most efficient ways to obtain a subset of properties from a JavaScript object is through destructuring. For example:
const object = {
a: 1,
b: 2,
c: 3
};
const {
a,
b
} = object;
const picked = {
a,
b
};
console.log(picked);
In this snippet, we have an object with three properties: a, b, and c. By destructuring, we assign the values of a and b to their respective variables and then create a new object picked, resulting in {a: 1, b: 2}.
Destructuring can also be utilized in function parameters, like so:
const pick = ({
a,
b
}) => ({
a,
b
});
const picked = pick(object);
console.log(picked);
Here, we define a function pick that returns an object containing the specified properties from the argument passed to it.
Section 1.2: Leveraging Lodash's Pick Method
Another method to extract properties is by using Lodash’s pick function. Here’s how it works:
const picked = _.pick(object, ['a', 'b']);
console.log(picked);
In this example, we call pick with the target object and an array of property names, resulting in the same output as before.
Section 1.3: Using Array.prototype.reduce
The reduce method can also be utilized to collect properties from an object into a new one. Consider the following example:
const picked = ['a', 'b'].reduce((resultObj, key) => ({
...resultObj,
[key]: object[key]
}), {});
console.log(picked);
In this case, we initiate reduce on an array containing the property names. The accumulator resultObj gathers the selected properties into a new object, yielding the expected result.
Conclusion
In summary, extracting a subset of properties from a JavaScript object can be efficiently accomplished using destructuring, Lodash’s pick, or the reduce method. Each of these techniques provides a straightforward approach to manage object properties effectively.
Check out this video for three different methods to access properties in JavaScript objects.
This video explains how to search for values in deeply nested JavaScript objects, a common interview question.