Mastering the Open-Closed Principle for Clean React Components
Written on
Understanding SOLID Principles
The SOLID principles serve as essential guidelines for software developers who prioritize the quality and maintainability of their code. While React is not inherently object-oriented, the core concepts of these principles can be beneficial. This article aims to illustrate how to apply these principles effectively for improved coding practices.
Previously, we explored the Single Responsibility Principle. Now, we turn our attention to the second principle of SOLID: the Open-Closed Principle.
What Is the Open-Closed Principle?
Thorben Janssen from Stackify articulates the Open-Closed Principle as follows:
“Robert C. Martin deemed this principle the ‘most crucial principle of object-oriented design.’ However, he was not the first to define it; Bertrand Meyer discussed it in 1988 in his book Object-Oriented Software Construction, explaining that:
‘Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.’”
In essence, this principle encourages developers to write code that allows for the addition of new functionality without altering existing code.
Applying the Principle: An Example
Imagine we have a User component designed to display a user's details.
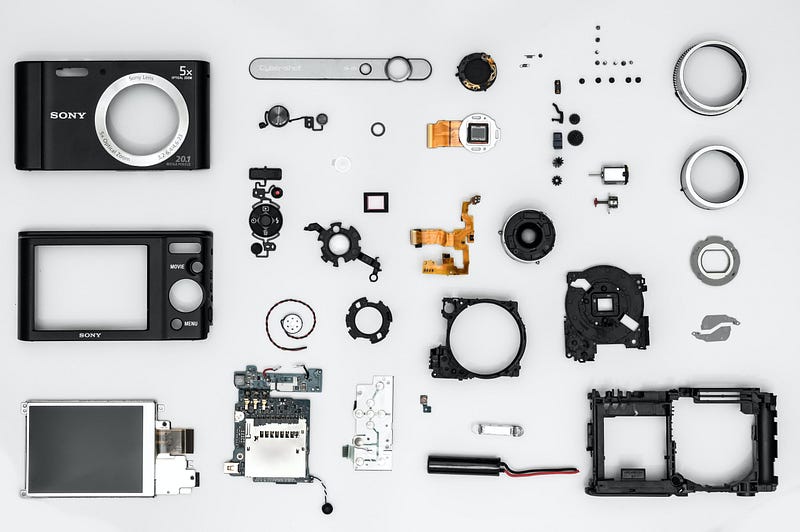
Initially, this seems straightforward. However, complexities arise when our manager informs us of three different user types in our system: SuperAdmin, Admin, etc., each requiring distinct information and functionalities.
A Misguided Approach
The most immediate solution might seem to involve adding conditional statements within our component to render different information based on user types.
Yet, this approach leads to several issues:
- The code becomes convoluted.
- Introducing a new user type necessitates modifying the User.js file, contradicting the Open-Closed Principle by requiring changes to existing code.
A Better Solution
To adhere to the Open-Closed Principle, we can utilize two main techniques: Higher-Order Components (HOCs) or Component Composition. While HOCs can be useful, component composition is often the preferred method recommended by Facebook.
#### Creating Dedicated User Components
To avoid conditionals in User.js, we can create distinct components for each user type:
- SuperAdmin Component
- Admin Component
Now, our App.js file might look something like this:
// Example of App.js with dedicated user components
This design allows us to add as many user types as necessary without revisiting existing code for modifications. Although some may argue this increases the number of files, it significantly enhances maintainability as the application scales.
A Word of Caution
While the SOLID principles are valuable, they should not be applied rigidly in every situation. As an experienced developer, it is crucial to strike a balance between code complexity and readability. Overemphasizing these principles can lead to "Too Much SOLID." It’s essential to recognize when these practices are beneficial versus when they may complicate your code unnecessarily.
Conclusion
Understanding and applying these principles can greatly enhance your coding journey. At the end of the day, well-structured code is what matters, and there’s no one-size-fits-all approach to programming.
Have a wonderful day!
The next principle, the Liskov Substitution Principle, is explored in detail here:
Applying the Liskov Substitution Principle in React
This video elucidates clean coding practices using the SOLID principles, particularly focusing on React applications.
In this video, viewers will gain insights into the SOLID principles and how they apply to writing clean code in a React.js application.