Exploring Software Design Patterns: A Comprehensive Overview
Written on
Chapter 1: Understanding Design Patterns
Design patterns represent standard, reusable solutions to frequently encountered issues in software design. It's crucial to note that design patterns are not ready-to-use code snippets; instead, they serve as templates or concepts to help address problems across various scenarios. When applied appropriately, they significantly enhance software efficiency by utilizing strategies that have been refined by countless developers.
Section 1.1: Benefits of Using Design Patterns
Employing design patterns in software development offers numerous advantages:
- Proven Solutions: Many developers have used these patterns, ensuring their effectiveness. They have undergone multiple revisions and optimizations.
- Reusability: Design patterns document adaptable solutions that can be tailored to address a variety of specific issues, as they are not tied to a single problem.
- Expressiveness: They allow for the elegant explanation of complex solutions.
- Communication: Familiarity with design patterns among developers facilitates clearer discussions about potential solutions.
- Reduced Refactoring: Utilizing the appropriate design pattern can minimize the need for future code refactoring.
- Compact Codebase: These patterns typically result in more streamlined, well-documented solutions that require less code.
Section 1.2: The Origins of Design Patterns
The foundational text on design patterns, “Design Patterns: Elements of Reusable Object-Oriented Software,” was published in 1994 by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, collectively known as the Gang of Four (GoF). Although the book primarily addresses C++, its principles are applicable to Java, JavaScript, Python, and other object-oriented languages. The authors identified 23 recurring patterns while developing large-scale enterprise systems, which can be utilized in various programming languages.
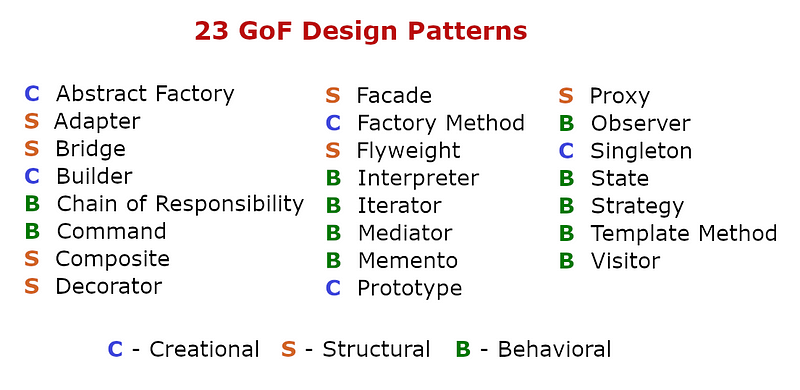
Chapter 2: The 23 Gang of Four Design Patterns
The 23 design patterns introduced by the Gang of Four are categorized into three groups:
Creational Patterns
These patterns focus on object creation mechanisms, allowing for flexible object instantiation. The five creational patterns are:
- Abstract Factory: Enables the creation of families of related objects without specifying their concrete classes.
- Builder: Separates the construction of a complex object from its representation, allowing for step-by-step object creation.
- Factory Method: Transfers the responsibility of object instantiation to a factory class, which generates instances of derived classes.
- Prototype: Offers a mechanism for cloning existing objects to create new ones, thus avoiding costly instantiation.
- Singleton: Ensures a class has only one instance and provides a global point of access to it.
Structural Patterns
These patterns address the composition of classes and objects, focusing on how they can work together. The seven structural patterns include:
- Adapter: Facilitates interaction between two incompatible interfaces.
- Bridge: Decouples abstraction from implementation, allowing both to evolve independently.
- Composite: Composes objects into tree structures to represent part-whole hierarchies.
- Decorator: Adds new functionality to objects dynamically without altering their structure.
- Facade: Provides a simplified interface to a complex subsystem.
- Flyweight: Minimizes memory usage by sharing common parts of objects.
- Proxy: Acts as an intermediary for another object to control access and reduce complexity.
Behavioral Patterns
These patterns focus on communication between objects. The eleven behavioral patterns include:
- Chain of Responsibility: Passes requests along a chain of handlers until one processes it.
- Command: Encapsulates a command request as an object, enabling parameterization and queuing.
- Interpreter: Implements a language's grammar and provides an interpreter for it.
- Iterator: Provides a way to access elements in a collection sequentially without exposing its internal structure.
- Mediator: Centralizes communication between objects to promote loose coupling.
- Memento: Captures an object's state to enable restoration of previous states.
- Observer: Notifies subscribers of changes in state to maintain consistency.
- State: Alters an object's behavior when its internal state changes.
- Strategy: Defines a family of algorithms and allows the selection of an algorithm at runtime.
- Template Method: Specifies the skeleton of an algorithm, deferring some steps to subclasses.
- Visitor: Separates an algorithm from the object structure it operates on, allowing for new operations without modifying the structure.
The first video, "Software Design Patterns, Principles, and Best Practices," provides a comprehensive exploration of design patterns, their principles, and best practices for effective software development.
The second video, "Introduction to Design Patterns in 25 Minutes," offers a quick yet informative overview of design patterns, making it a great starting point for beginners.
Conclusion
By now, you should have a foundational understanding of essential software design patterns utilized across various programming languages. To develop your skills further, consider implementing these patterns in your projects or practicing them in coding exercises. While design patterns can enhance many implementations, they should not be seen as quick fixes for every scenario. Genuine problem-solving ability in software engineering remains irreplaceable. Recognizing design patterns provides a common language for conceptualizing recurring issues and solutions, especially when collaborating with teams or managing extensive codebases. It's vital for developers to grasp the reasoning behind each design pattern thoroughly.
Feel free to connect with me through my personal newsletter/blog, LinkedIn, Twitter, and GitHub.