Maximize Your Python Code Efficiency with These 10 Tips
Written on
Best Practices for Efficient Python Programming
Python is celebrated for its ease of use and clarity, making it a favored choice among developers. However, crafting efficient Python code involves more than just familiarity with its syntax. By adhering to established best practices, you can enhance both the performance and readability of your code. This article will delve into 10 essential practices for efficient Python programming, aimed at helping you refine your coding skills.
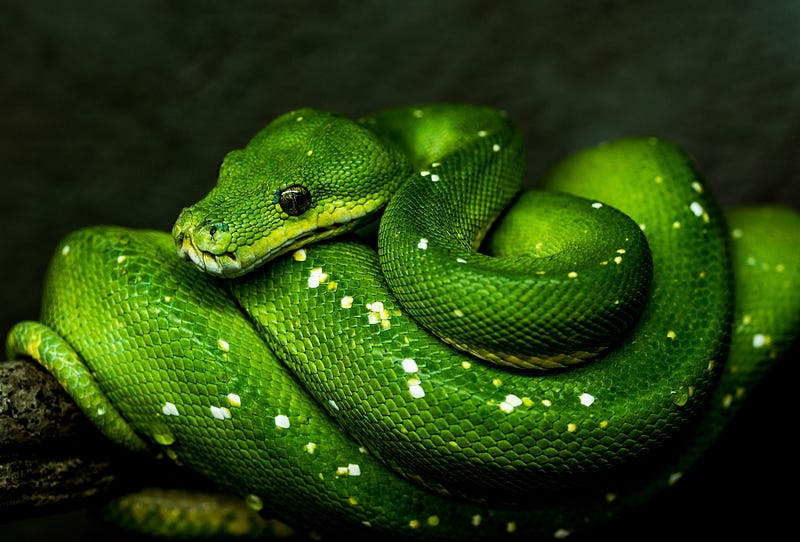
Utilize Built-in Functions and Libraries
Python provides an extensive array of built-in functions and standard libraries that can assist you in accomplishing tasks more effectively. Before creating your own solutions, check for existing built-in options that may suit your needs. Leveraging these resources can save time, minimize errors, and enhance code clarity.
Avoid Global Variables
Using global variables can complicate your code, making it less understandable and harder to maintain. They can lead to unintended side effects and reduce the modularity of your functions. Instead, consider passing variables as arguments to functions or utilizing classes to encapsulate states.
Embrace List Comprehensions
List comprehensions offer a streamlined approach to generating lists in Python. They can outperform traditional loops, particularly when dealing with extensive data sets. Implementing list comprehensions can lead to more succinct and efficient code.
Incorporate Generators
Generators are a robust feature in Python that enables iteration over a sequence without needing to create a complete list in memory. This is particularly beneficial when handling large data sets or generating infinite sequences. Employing generators can enhance memory efficiency and performance.
Profile Your Code
Before embarking on code optimization, it’s crucial to profile your code to uncover performance bottlenecks. Python’s built-in cProfile module, along with other tools like Py-Spy, can assist in analyzing your code’s performance and identifying areas needing improvement.
Optimize Loop Performance
Loops often present performance challenges in Python applications. To optimize them, consider using built-in functions such as map() and filter(), or stick to list comprehensions. Additionally, refrain from using the range() function with extensive ranges, as it may consume substantial memory.
Select Appropriate Data Structures
Choosing the right data structure for your specific requirements can greatly influence your code’s efficiency. Python offers various built-in data structures like lists, tuples, sets, and dictionaries, each with unique advantages and disadvantages. Recognizing when to apply each data structure can lead to more effective coding.
Cache Results via Memoization
Memoization is a strategy that involves storing the results of costly function calls and returning the cached result for repeated inputs. This technique can help you avoid unnecessary computations and enhance performance. The functools module in Python includes an lru_cache decorator that simplifies the implementation of memoization in your functions.
Utilize the timeit Module for Performance Testing
When assessing the performance of different code implementations or snippets, turn to Python’s built-in timeit module. This module provides a straightforward and precise method for measuring the execution time of your code, allowing for effective comparison of various solutions.
Prioritize Code Readability
Enhancing your code’s performance should not compromise its readability. Aim to write clear, concise, and well-documented code that is easy for others to understand and maintain. Remember, code is often read more frequently than it is written, so it’s vital to balance readability with efficiency.
Conclusion
Crafting efficient Python code transcends merely making it functional. By adhering to these 10 best practices, you can optimize your code for performance, readability, and ease of maintenance. As you continue to develop your Python skills, keep in mind that the essence of efficient coding lies in understanding the language’s capabilities, utilizing the right tools, and maintaining clarity. With these guiding principles, you’ll be on your path to becoming a more skilled and effective Python developer.
Additional Resources
This video, titled "Your Python Code NEEDS These 5 EASY Optimizations," discusses essential techniques to enhance your Python programming practices.
In this video, "How Sets Can Truly OPTIMIZE Your Python Code," you'll discover how using sets can improve the efficiency of your code.