Understanding the JSON.parse() Method: Your Guide to Parsing JSON Data
Written on
Chapter 1: Introduction to JSON
JSON, or JavaScript Object Notation, is a simple and lightweight format for data interchange that is both human-readable and writable. In JavaScript, the JSON.parse() method is essential for converting a JSON string into a JavaScript object. This guide will delve into the functionality of JSON.parse(), its syntax, and include practical coding examples for a comprehensive understanding.
Section 1.1: What is the JSON.parse() Method?
The JSON.parse() method in JavaScript is utilized to interpret a JSON string and transform it into a JavaScript object. By accepting a valid JSON string as an input, it returns the corresponding JavaScript object. This method is especially useful when handling data received from a server or stored as a string.
Subsection 1.1.1: Syntax of JSON.parse()
The syntax for the JSON.parse() method is straightforward:
JSON.parse(text[, reviver])
- text: The JSON string you wish to parse into a JavaScript object.
- reviver (optional): A function that can modify the results. This parameter is not commonly used in basic applications.
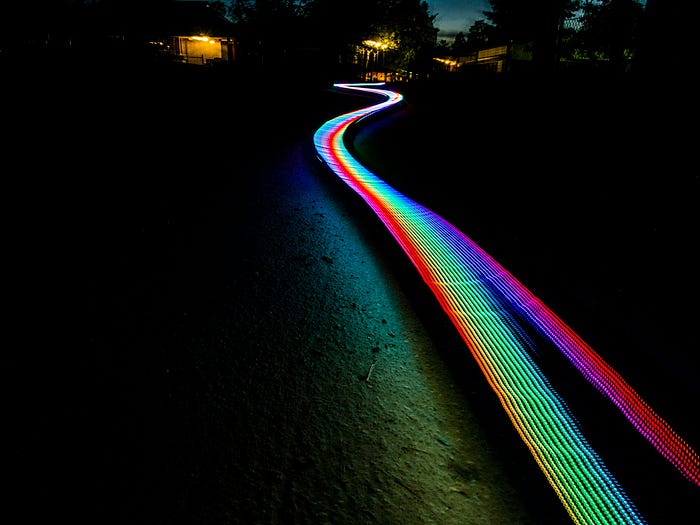
Section 1.2: Utilizing JSON.parse()
To implement the JSON.parse() method, you simply provide a valid JSON string as an argument. Here’s a practical example:
const jsonString = '{"name": "John", "age": 30}';
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Output: John
console.log(jsonObject.age); // Output: 30
In this scenario, the JSON string denotes an object with properties for name and age. By invoking JSON.parse(jsonString), we convert the string into a JavaScript object, allowing us to access its properties as we would with any regular object.
Chapter 2: Error Handling with JSON.parse()
When using JSON.parse(), it is crucial to manage potential errors, particularly with invalid JSON strings. If the string is not valid JSON, a SyntaxError will be thrown. To handle this error gracefully, you can use a try-catch block:
const invalidJsonString = '{name: "John", age: 30}';
try {
const jsonObject = JSON.parse(invalidJsonString);
console.log(jsonObject);
} catch (error) {
console.error('Invalid JSON string:', error);
}
By encapsulating the JSON.parse() call within a try-catch block, you can effectively handle any parsing errors that may arise.
Section 2.1: Real-World Application
Let’s examine a real-world scenario where we fetch JSON data from an API and parse it using JSON.parse():
.then(response => response.json())
.then(data => {
const parsedData = JSON.parse(data);
console.log(parsedData);
})
.catch(error => console.error('Error fetching data:', error));
In this example, we first retrieve data from an API using the Fetch API, convert the response to JSON with .json(), and subsequently parse the JSON data using JSON.parse().
Conclusion
In summary, the JSON.parse() method is a vital tool in JavaScript for managing and processing JSON data. By familiarizing yourself with its syntax and application, you can efficiently work with JSON strings and convert them into JavaScript objects. Always remember to handle errors and consider utilizing the optional reviver function for more complex cases. Begin experimenting with various JSON strings to see how JSON.parse() can enhance your data handling tasks.