Creating a Simple Video Player in React Native with Expo
Written on
Chapter 1: Introduction to React Native Video Player
In this guide, we will explore the steps necessary to construct a straightforward video player in React Native using Expo. This tutorial will walk you through the entire process, ensuring that your video player functions seamlessly on both Android and iOS platforms.
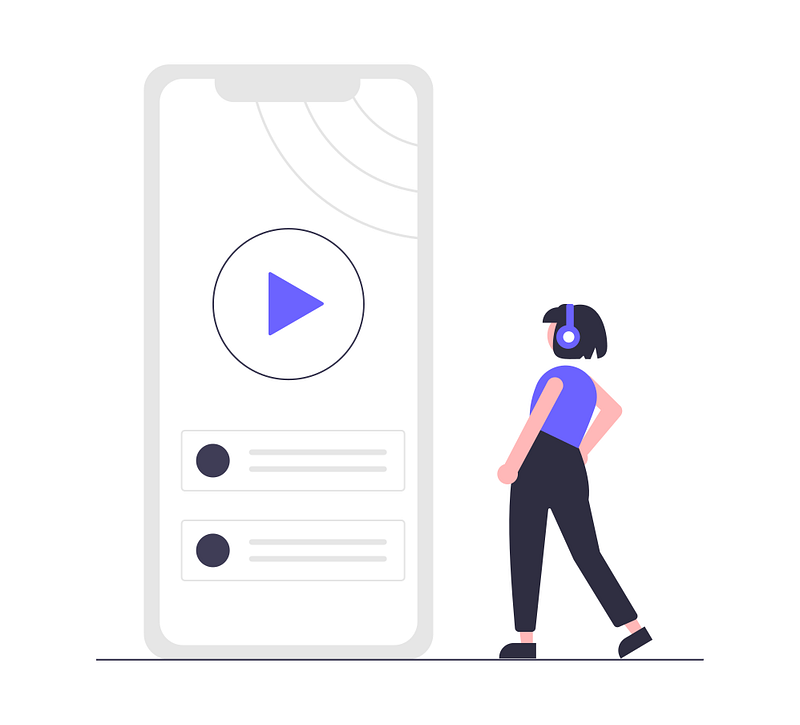
Creating an Expo Project
To start, we need to set up a new project using the Expo CLI. Open your terminal and enter the following command (make sure you're in the desired directory):
expo init video-player
This command will prompt you to select a project template; you can choose either a blank JavaScript or a blank TypeScript project. For the purpose of this tutorial, we will select the blank TypeScript option. After a brief wait, your Expo project will be ready for development.
Once the installation completes, run the following command to launch your project in the Android or iOS simulator:
expo start
A menu will appear, allowing you to select your preferred simulator: press “a” for Android or “i” for iOS. After making your selection, the simulator will build and execute your project.
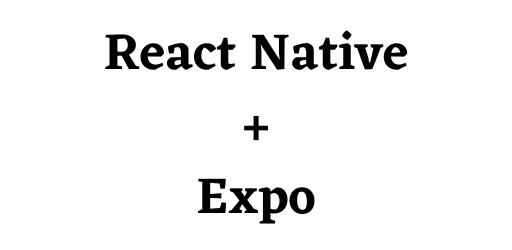
Setting Up the Video Component
After the project has been initialized, it’s time to incorporate the Video component. This component, part of the expo-av library, enables you to display videos alongside other UI elements within your application. To proceed, you must first install expo-av. Open your terminal at the project’s root path and run:
expo install expo-av
This command will add the necessary library to your project dependencies.
Next, navigate to the App.tsx file. You should see the following code snippet:
export default function App() {
return (
<View style={styles.container}>
<Text>Open up App.tsx to start working on your app!</Text>
<StatusBar style="auto" />
</View>
);
}
Remove the highlighted code lines, as we will be implementing our video player within the View tag.
Usage of the Video Component
First, import the Video component from the expo-av library:
import { Video } from "expo-av";
Now, you can include the Video component in your code. Place the Video component inside the View tag, ensuring to provide the necessary props.
#### Required Props
Source Prop
The source prop specifies the path to the video you wish to play. It is formatted as a URI:
<Video
source={{
uri: "http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4",}}
/>
Style Prop
To ensure the player is visible, you must provide the style prop, setting both width and height. Here’s an example:
<Video
style={styles.video}
source={{
uri: "http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4",}}
/>
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
video: {
alignSelf: 'center',
width: 320,
height: 200,
},
});
With these settings, you should now be able to see and play the video specified in the URI.
Optional Props for Enhanced Functionality
Resize Mode Prop
This prop determines how the video will scale within the component's dimensions. It can take the following values:
- Contain: Fits within the bounds while maintaining aspect ratio.
- Cover: Fills the bounds while preserving aspect ratio.
- Stretch: Fills the component bounds.
I typically prefer using contain:
<Video
style={styles.video}
resizeMode="contain"
source={{
uri: "http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4",}}
/>
Use Native Controls Prop
This boolean prop enables native playback controls (like play and pause) within the Video component:
<Video
style={styles.video}
resizeMode="contain"
source={{
uri: "http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4",}}
useNativeControls={true}
/>
Is Looping Prop
Set this boolean to define whether the video should loop indefinitely (true) or play just once (false):
<Video
style={styles.video}
resizeMode="contain"
source={{
uri: "http://d23dyxeqlo5psv.cloudfront.net/big_buck_bunny.mp4",}}
useNativeControls={true}
isLooping={true}
/>
Poster Source Prop
While the video loads, you can display a placeholder image to inform users that loading is in progress. This enhances user experience.
Although there are additional useful props associated with the Video component, this guide has covered the essential features necessary for a basic video player capable of playing videos from local storage or the web.
For further details about the props available for the Video component, refer to the official documentation:
Video - Expo Documentation
The Video component from Expo allows for inline video display alongside other UI elements in your application.
The first video titled "How to Add Videos to your Expo React Native Apps" provides a comprehensive overview of integrating video functionality in your projects.
The second video, "React Native - Video Player with Caching using Expo (Part 1/6)," delves into more advanced features for video playback in React Native apps.
Conclusion
You can download the complete project from [this link](#). Thank you for taking the time to read this tutorial. If you found the information helpful, please consider following me and leaving a clap or comment to support the article.
If you enjoy this type of content and would like to support my work as a programmer and writer, consider signing up for Medium with my link for unlimited access to various articles and tutorials.