Utilizing Laplace Transforms in Python for Differential Equations
Written on
Chapter 1: Introduction to Laplace Transforms
In our previous discussion, we delved into the concept of Laplace transforms and their inverses using Python. Today, we will apply this knowledge to tackle differential equations. Let's consider the following differential equation:

This equation models a forced oscillator experiencing friction in the realm of physics. For our initial conditions, we will set (y(0) = y'(0) = 0).
The Role of Laplace Transforms
The Laplace transform serves as a highly effective technique for solving such equations. To illustrate, let’s observe what occurs when we apply the Laplace transform to the second derivative of our unknown function:
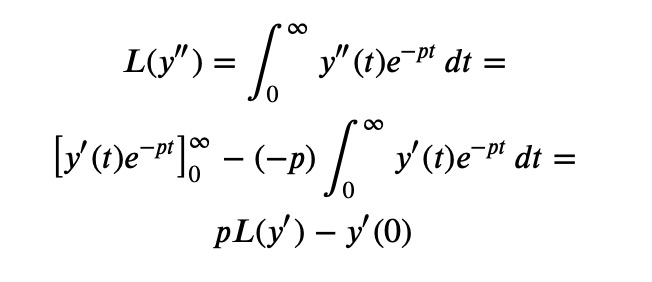
Here, we have utilized partial integration to transition from the first to the second line. We can express the second derivative by multiplying it by (p) and then adjusting for the initial condition of the first derivative. Similarly, we can handle (y'(t)) to obtain:

Thus, we arrive at:

This allows us to apply the Laplace transform to the entire differential equation. Now, let’s switch gears and open a Jupyter notebook to initiate our work in Python. We need to define our symbols and the differential equation, along with the unevaluated Laplace transform:
from sympy import *
t, p = symbols('t, p')
y = Function('y')
# The unevaluated Laplace transform:
Y = laplace_transform(y(t), t, p)
eq = Eq(diff(y(t), (t, 2)) + 2 * diff(y(t), t) + 16*y(t),
cos(4*t))
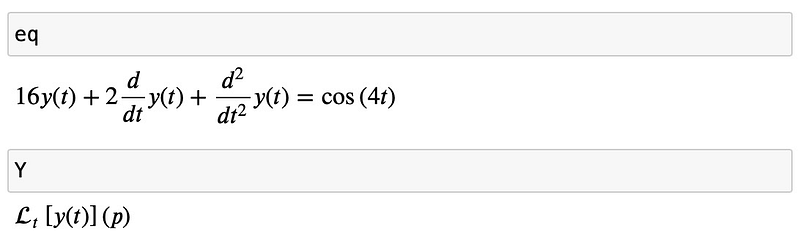
The right-hand side of the equation appears as follows:

But how do we express this in Sympy? The Subs class can be utilized! This class represents unevaluated substitutions of an expression, which is precisely what we need. Thus, our initial conditions can be represented as:

Now, we can write the Laplace transform of the differential equation as:
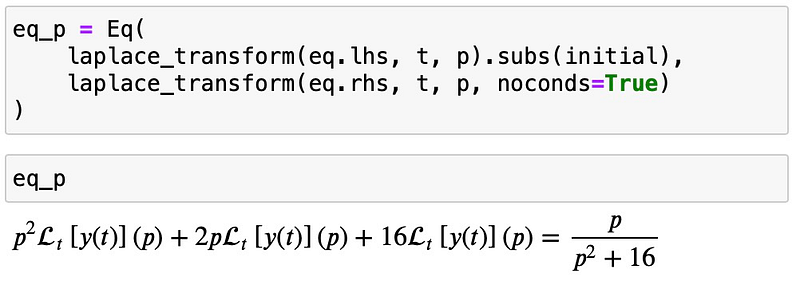
Next, let’s solve for (L[y]):
![Solving for L[y]](https://batteriesinfinity.com/all_images/2024-09-25/65578_11.jpg)
and revert from Laplace space back to the normal domain:
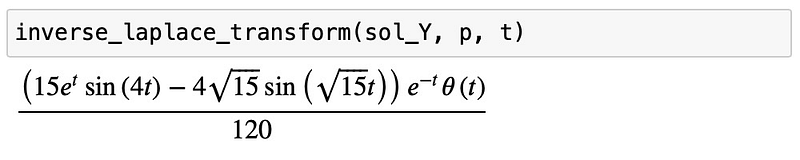
We can then tidy up our result:
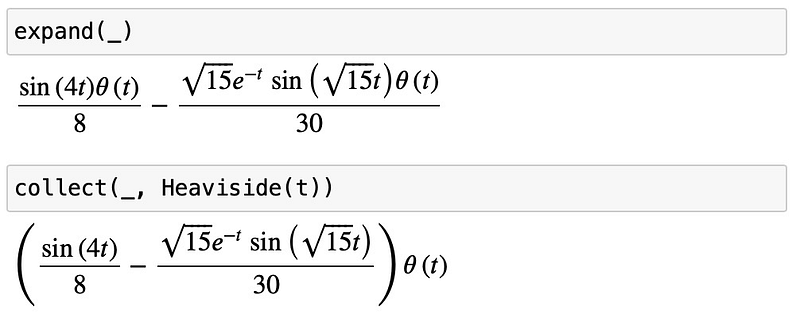
This gives us a clean representation where the Heaviside function ensures all values with (t < 0) are zero, which is acceptable since we are only interested in solutions for (t geq 0). It’s noteworthy that the Laplace method inherently handles initial conditions, sparing us the complexity of determining constants from a general solution. This feature makes it significantly more user-friendly compared to many other methods.
Finally, let's visualize the solution:
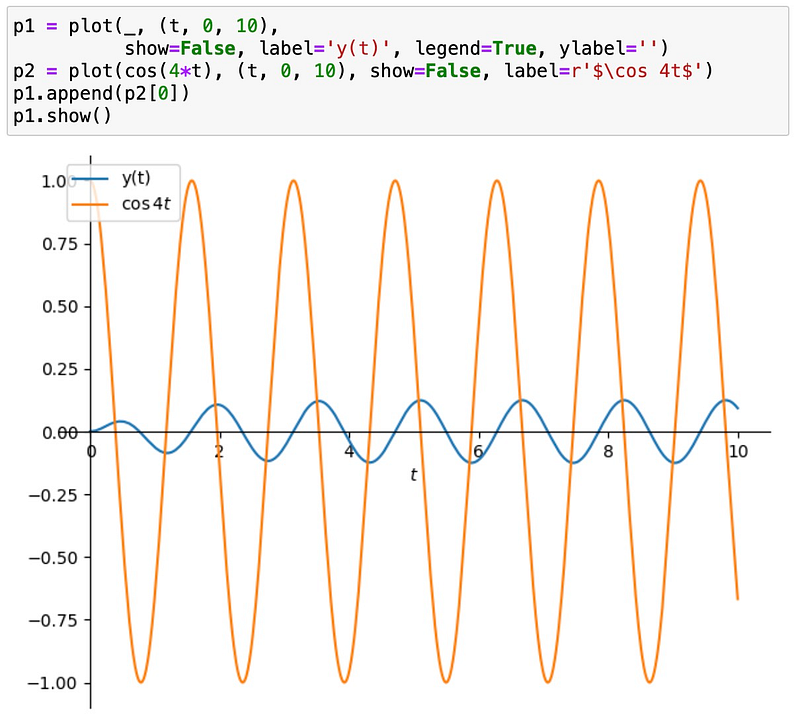
As illustrated, the solution (in blue) takes some time to respond to the external force. Eventually, it oscillates at the same frequency but with a persistent phase shift.
Extending Beyond Homogeneous Initial Conditions
The example provided demonstrates solving a problem with homogeneous initial conditions ((y(0) = y'(0) = 0)). However, the Laplace technique is not confined to this scenario. By simply inputting non-homogeneous initial conditions, solving for (y(t)), and performing the inverse Laplace transform, you can readily obtain the solution (y(t)).
Chapter 2: Video Resources
To further enhance your understanding of Laplace transforms and their applications in solving differential equations, check out the following videos:
This video covers the concept of Laplace Transforms using Sympy.
In this video, learn how to solve ordinary differential equations with Laplace Transforms.